Microsoft develops samples and documentation that follow the guidelines in this topic. If you follow the same coding conventions, you may gain the following benefits:
Your code will have a consistent look, so that readers can better focus on content, not layout.
Readers understand your code more quickly because they can make assumptions based on previous experience.
You can copy, change, and maintain the code more easily.
You help ensure that your code demonstrates 'best practices' for Visual Basic.
Microsoft Open Source Code of Conduct. This project has adopted the Microsoft Open Source Code of Conduct. For more information see the Code of Conduct FAQ or contact opencode@microsoft.com with any additional questions or comments. Data and telemetry. This extension collects usage data and sends it to Microsoft to help improve our products. One place for all extensions for Visual Studio, Azure DevOps Services, Azure DevOps Server and Visual Studio Code. Discover and install extensions and subscriptions to create the dev environment you need.
Naming Conventions
For information about naming guidelines, see Naming Guidelines topic.
Do not use 'My' or 'my' as part of a variable name. This practice creates confusion with the
My
objects.You do not have to change the names of objects in auto-generated code to make them fit the guidelines.
Layout Conventions
Insert tabs as spaces, and use smart indenting with four-space indents.
Use Pretty listing (reformatting) of code to reformat your code in the code editor. For more information, see Options, Text Editor, Basic (Visual Basic).
Use only one statement per line. Don't use the Visual Basic line separator character (:).
Avoid using the explicit line continuation character '_' in favor of implicit line continuation wherever the language allows it.
Use only one declaration per line.
If Pretty listing (reformatting) of code doesn't format continuation lines automatically, manually indent continuation lines one tab stop. However, always left-align items in a list.
Add at least one blank line between method and property definitions.
Commenting Conventions
Put comments on a separate line instead of at the end of a line of code.
Start comment text with an uppercase letter, and end comment text with a period.
Insert one space between the comment delimiter (') and the comment text.
Do not surround comments with formatted blocks of asterisks.
Program Structure
When you use the
Main
method, use the default construct for new console applications, and useMy
for command-line arguments.
Language Guidelines
String Data Type
Use string interpolation to concatenate short strings, as shown in the following code.
To append strings in loops, use the StringBuilder object.
Relaxed Delegates in Event Handlers
Do not explicitly qualify the arguments (Object and EventArgs) to event handlers. If you are not using the event arguments that are passed to an event (for example, sender as Object, e as EventArgs), use relaxed delegates, and leave out the event arguments in your code:
Microsoft Visual Code 64
Unsigned Data Type
- Use
Integer
rather than unsigned types, except where they are necessary.
Arrays
Use the short syntax when you initialize arrays on the declaration line. For example, use the following syntax.
Do not use the following syntax.
Put the array designator on the type, not on the variable. For example, use the following syntax:
Do not use the following syntax:
Use the { } syntax when you declare and initialize arrays of basic data types. For example, use the following syntax:
Do not use the following syntax:
Use the With Keyword
When you make a series of calls to one object, consider using the With
keyword:
Use the Try..Catch and Using Statements when you use Exception Handling
Do not use On Error Goto
.
Use the IsNot Keyword
Use the IsNot
keyword instead of Not..Is Nothing
.
New Keyword
Use short instantiation. For example, use the following syntax:
The preceding line is equivalent to this:
Use object initializers for new objects instead of the parameterless constructor:
Event Handling
Use
Handles
rather thanAddHandler
:Use
AddressOf
, and do not instantiate the delegate explicitly:When you define an event, use the short syntax, and let the compiler define the delegate:
Do not verify whether an event is
Nothing
(null) before you call theRaiseEvent
method.RaiseEvent
checks forNothing
before it raises the event.
Using Shared Members
Call Shared
members by using the class name, not from an instance variable.
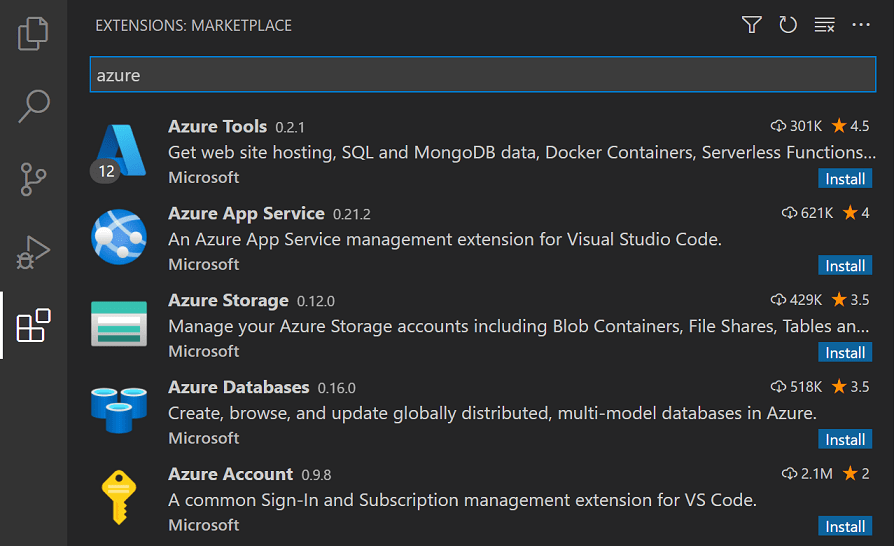
Use XML Literals
XML literals simplify the most common tasks that you encounter when you work with XML (for example, load, query, and transform). When you develop with XML, follow these guidelines:
Use XML literals to create XML documents and fragments instead of calling XML APIs directly.
Import XML namespaces at the file or project level to take advantage of the performance optimizations for XML literals.
Use the XML axis properties to access elements and attributes in an XML document.
Use embedded expressions to include values and to create XML from existing values instead of using API calls such as the
Add
method:
LINQ Queries
Use meaningful names for query variables:
Provide names for elements in a query to make sure that property names of anonymous types are correctly capitalized using Pascal casing:
Rename properties when the property names in the result would be ambiguous. For example, if your query returns a customer name and an order ID, rename them instead of leaving them as
Name
andID
in the result:Use type inference in the declaration of query variables and range variables:
Align query clauses under the
From
statement:Use
Where
clauses before other query clauses so that later query clauses operate on the filtered set of data:Use the
Join
clause to explicitly define a join operation instead of using theWhere
clause to implicitly define a join operation:
See also
Download Visual Studio Code For Windows 10
Notice

Some of the downloads that are mentioned in this article are currently available on My.VisualStudio.com. Make sure to log in by using a Visual Studio Subscription account so that you can access the download links.
If you are asked for credentials, use your existing Visual Studio subscription account or create a free account by selecting 'Create a new Microsoft account.'
Summary
This article lists the download links for the latest versions of Microsoft Visual C++.
Visual Studio 2015, 2017 and 2019
Download the Microsoft Visual C++ Redistributable for Visual Studio 2015, 2017 and 2019. The following updates are the latest supported Visual C++ redistributable packages for Visual Studio 2015, 2017 and 2019. Included is a baseline version of the Universal C Runtime see MSDN for details.
x86: vc_redist.x86.exe
x64: vc_redist.x64.exe
ARM64: vc_redist.arm64.exe
Note Visual C++ 2015, 2017 and 2019 all share the same redistributable files.
For example, installing the Visual C++ 2019 redistributable will affect programs built with Visual C++ 2015 and 2017 also. However, installing the Visual C++ 2015 redistributable will not replace the newer versions of the files installed by the Visual C++ 2017 and 2019 redistributables.
This is different from all previous Visual C++ versions, as they each had their own distinct runtime files, not shared with other versions.
Visual Studio 2013 (VC++ 12.0)
Download the Microsoft Visual C++ Redistributable Packages for Visual Studio 2013. This is the latest supported Visual C++ redistributable package for Visual Studio 2013.
Download Multibyte MFC Library for Visual Studio 2013. This add-on for Visual Studio 2013 contains the multibyte character set (MBCS) version of the Microsoft Foundation Class (MFC) Library.
Download Visual C++ 2013 Runtime for Sideloaded Windows 8.1 apps.
For more information, see C++ Runtime for Sideloaded Windows 8.1 apps on the VC++ Team Blog.
Visual Studio 2012 (VC++ 11.0)
Download the Microsoft Visual C++ Redistributable Packages for Visual Studio 2012 Update 4. This is the latest supported Visual C++ redistributable package for Visual Studio 2012.
Visual Studio 2010 (VC++ 10.0) SP1
Microsoft Visual Code Ubuntu
Download the Visual Studio 2010 Service Pack 1 (Installer). This is the latest supported Visual C++ service pack for Visual Studio 2010.
Note: This web installer requires an internet connection. This installer downloads and installs Visual Studio 2010 Service Pack 1. It works for all editions of Visual Studio 2010 (Express, Professional, Premium, Ultimate, and Test Professional).
Download the Microsoft Visual C++ 2010 Service Pack 1 Redistributable Package MFC Security Update. This is the latest supported Visual C++ redistributable package update for Visual Studio 2010.
Microsoft Visual Code Studio Download
Visual Studio 2008 (VC++ 9.0) SP1
Visual Studio 2008 reached end of support on April 10, 2018. To aid the discovery of the latest downloads, the links are retained currently, but may be removed in the future.
Download the Visual Studio 2008 Service Pack 1 (Installer). This is the latest Visual C++ service pack for Visual Studio 2008. This service pack improved responsiveness, stability, and performance.
Note This download installs Visual Studio 2008 Service Pack 1 and Microsoft .NET Framework 3.5 SP1.Download the Microsoft Visual C++ 2008 Service Pack 1 Redistributable Package MFC Security Update. This is the latest Visual C++ redistributable package update for Visual Studio 2008.
Microsoft Visual Code Ssh
Visual C++ Redistributable Packages
Visual C++ Redistributable Packages install runtime components of Visual C++ Libraries on a computer that does not have Visual C++ installed. The libraries are required to run applications that are developed by using the corresponding version of Visual C++.
For Visual Studio 2008
These packages install runtime components of C Runtime (CRT), Standard C++, ATL, MFC, OpenMP and MSDIA libraries. They are installed into the native assembly cache, also known as the WinSxS folder. They are installed on versions of Windows operating systems that support side-by-side assemblies, for libraries that support side-by-side deployment models (CRT, STL, ATL, MFC, OpenMP).
Angry birds wm 6.5.
Microsoft Foundation Class Library Security Update
A security issue was identified. This issue causes a Microsoft Foundation Class Library application vulnerability in your Windows-based system that uses the Visual C++ Redist. The Microsoft Foundation Class Library Security Update packages in this article have the most current redistributable files for Visual Studio.
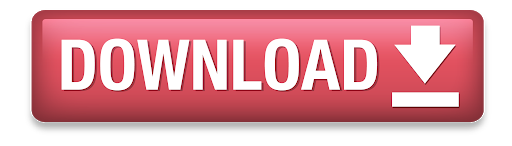